Pythonのフレームワーク Kivy を利用した比較的簡単な機能の追加についてまとめる。今回扱う機能(Widget(ウィジェット))は TextInput(テキストインプット)である。
実装は下記のステップで行う。
- step1:
- step2:
- step3:
- step4:
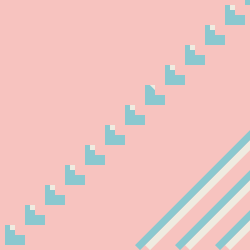
プログラムの構成
- Pythonコード
- Kivyコード
- Python自作ライブラリー
1. Python: main_textinput_s.py
# -*- coding: utf-8 -*-
# - - - - - - - - - - - - - - (Kivy)
import os
import japanize_kivy # kvファイル内で日本語を扱えるようにする
from kivy.app import App
# from kivy.core.window import Window
from kivy.config import Config
Config.set("graphics", "width", "720")
Config.set("graphics", "height", "690") # 高さはタイトルバー(30px)分だけ引いておくと想像の大きさと一致する
from kivy.uix.screenmanager import ScreenManager, Screen, NoTransition, SlideTransition
from kivy.properties import StringProperty, NumericProperty, ObjectProperty
from kivy.uix.widget import Widget #
from kivy.uix.label import Label # #003
from kivy.uix.button import Button # #004
from kivy.uix.textinput import TextInput # #005
# グローバル変数の定義
text_window_title: str = "study_widget" # window上部のタイトルバーに表示する文字列
list_menu_tag: list = ['MainScreen', 'sub'] # 生成する予定の画面の名前(プログラム上で使用する名前)
# ---自作関数の定義---------------------------------------------- #
def test():
print("test():自作関数test()が呼び出されました(普通の定義)")
#- - - - - - - - kivyオブジェクトの流用するための設定 - - - - - - - - -#
# - - Screen の設定を継承した設定を定義 - - -
class MyScreen(Screen):
# python内で変数や関数の設定を追加変更しない。default状態。
pass
# - - MyScreen の設定を継承した Screen の設定を定義 - - -
class MainScreen(MyScreen):
pass
class sub(Screen):
pass
class MyTextInput(TextInput):
pass
# kivyスクリプト内で呼び出す変数
path_image = StringProperty(f"{os.path.dirname(__file__)}"+r"\elements\background_active_image_1.png")
# GUIのクラス定義:スクリーンをGUI内部でインスタンス化
# ここのクラス名はkvファイルの名前と同一にすること: gui_textinput_s.kv
# App はアプリケーションそのもの
class gui_textinput_s(App):
global text_window_title
global list_menu_tag
# アプリの初期化設定
def __init__(self, **kwargs):
super(gui_textinput_s, self).__init__(**kwargs)
self.title = text_window_title
print("initiarized!")
# アプリの内部構造設定: 今回はスクリーンマネージャーのみを組み込む
def build(self):
global object_sm
self.sm = ScreenManager(transition=NoTransition()) # アニメーションなしで画面を瞬時に切り替える設定
self.sm.add_widget(MainScreen(name=list_menu_tag[0])) # 各スクリーンに名前をつけて管理する
self.sm.add_widget( sub(name=list_menu_tag[1])) # それらの名前の画面(Screen)をアプリ内に追加する
print(f"今表示している画面は{self.sm.current_screen}")
print("built!")
return self.sm
# ---機能上のメイン処理----------------------------------------------- #
def main():
gui_textinput_s().run()
# ----------------------------------------- application_start
if __name__ == '__main__':
main()
# ----------------------------------------- application_end__
# ------------------------------------------------------------------- #
print("done!")
# - - - - - - - - - - - - - - - - - - - - - - - - - -
# end_of_file: this line is 84
2. Kivy: gui_textinput_s.kv
#: kivy 2.1.0
#: import get_color_from_hex kivy.utils.get_color_from_hex
<MyScreen>:
# 画面の背景色設定
canvas.before:
Color:
rgba: get_color_from_hex('#193549ff') #E6E4E0FF
Rectangle:
size: self.size
pos: self.pos
<MyTextInput>:
# プロパティ
text: "" # プレーンテキスト用のボックス(テキストインプット)内にデフォルトので表示するテキスト
halign: 'left' # 水平(横)方向の位置揃え: horizontal align(整列)
valign: 'center' # 鉛直(縦)方向の位置揃え: vertical align(整列)
width: "550px" # テキストインプットの横幅
height: "200px" # テキストインプットの縦幅
size_hint: (None,None) # テキストインプットの縦横比: Noneを指定することで上記横幅・縦幅が適用される
pandding: ( "0px", "0px")# border領域"の内側の余白を設定
margin: ( "0px", "0px")# border領域"の外側の余白を設定
pos: ("90px","400px")# テキストインプットの位置 (原点は左下)
font_size: "9px" # テキストの大きさ
multiline: False # デフォルト: True のとき Enterで改行される, Falseのときでは入力を終了する(改行不可能でフォーカスが外れる)
# 以上のプロパティ以外にもいくつも項目が存在する。
<MainScreen>:
Label:
id: label_main
font_size: "24px"
text: "main"
MyTextInput:
# プロパティ
id: mti_01
background_active: self.path_image
background_normal: r".\elements\background_nomal_image_1.png"
# イベント
on_double_tap:
print("on_double_tap occurred!") # ダブルタップのときに発生するイベント
on_triple_tap:
print("on_triple_tap occurred!") # トリプルタップのときに発生するイベント
# step2で追加する位置
▶step4で追加するコード
print(self.focus)
on_text_validate:
print("on_text_validate occurred!") # multiline=Flaseのときにのみ機能する(発生する)イベント
mti_02.focus = True
on_focus:
print("on_focus") # フォーカス(アクティブ、文字入力可能な)状態になったときに発生するイベント
MyTextInput:
# プロパティ
id: mti_02
pos: ("90px", "90px")# テキストインプットの位置 (原点は左下)
background_active: self.path_image
# イベント
on_text_validate:
print("on_text_validate occurred!") # multiline=Flaseのときにのみ機能する(発生する)イベント
mti_01.focus = True
TextInputに関する公式ドキュメント:
https://kivy.org/doc/stable/api-kivy.uix.textinput.html?highlight=on_focus
EOF